Design Principles
- Ergonomics ≠ abstraction: A better DX doesn't have to cost you control. We make both work together.
- Interop-first: (C & C++) ABI, bit-level layout control, and no runtime — Helix plays nice with everything else.
- Community-driven: We prioritize feedback from our users to continuously improve the language.
- Performance-focused: We ensure that the language is optimized for speed and efficiency.
- Documentation-rich: Comprehensive guides and examples to help you get started quickly.
fn fib(const n: i32) -> i32 { if n <= 1 { return n }
return fib(n - 1) + fib(n - 2)}
test "fib" { assert: fib(5) == 5 assert: fib(7) == 13}
Why Helix?
Most low-level languages force you to choose: either get raw power with cryptic syntax, or modern ergonomics with unpredictable performance. Helix gives you both.
- Zero-cost abstractions: Write clean code that compiles to exactly what you'd hand-write in assembly (if you had the time).
- Predictable performance: No hidden allocations. No background magic. What you see is what runs.
- Memory-first mindset: Manual control, optional ownership tracking, and a layout model that makes cache misses cry.
- Tooling built-in: From formatter to linter to package manager — you get batteries included, not duct-taped on.
- 0+
Weekly Downloads
- 0+
Total GitHub Stars
- 0+
Projects Use Helix
Mission
Helix exists to redefine how we write low-level software by combining the
raw power of systems programming with modern language design.
Our mission is to give developers fine-grained control over performance, memory,
and safety — without drowning them in complexity or sacrificing clarity.
We're building a language that scales from tiny embedded systems to high-performance
native applications, with predictable behavior and zero-cost abstractions by
default.
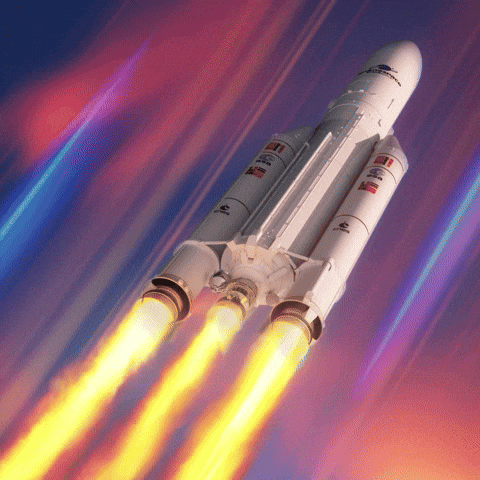
People who give us money! (or want to Donate)
The HSF is in the process of becoming a 501(c)(3) nonprofit organization in the near
future, building open-source tools that push the boundaries of systems
programming—while staying accessible, readable, and performant by default. Your
donations directly fund compiler research, language development, documentation, and
education efforts.
Whether you're a believer in better languages, a company that cares about sustainable
infrastructure, or just someone tired of chasing segfaults at 3am—supporting Helix is
your way of building the future of systems programming. Plus, it's tax-deductible. What's
not to love?
To support us, consider contacting
[email protected] to make a donation through our website or sharing our mission with your network. Every
bit helps us continue our work and improve the Helix language for everyone.
Thank you for considering supporting our mission!